Critical Tips to write Clean Code In C#
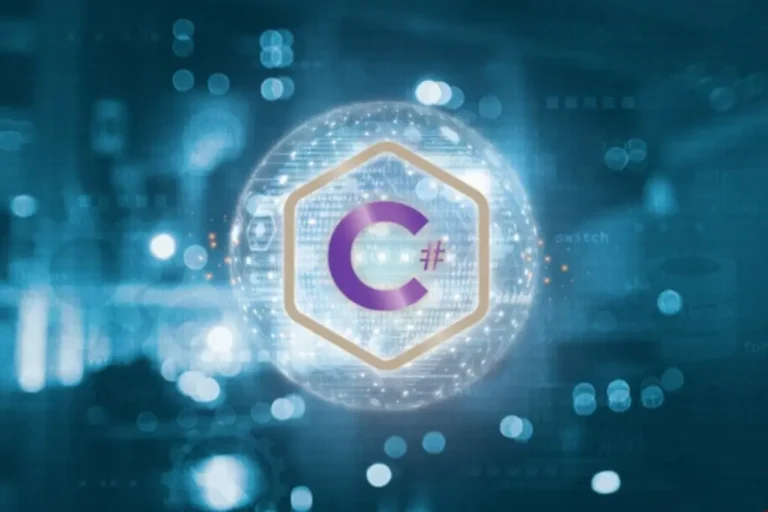
Any developer can write code with good training and practice. There would be many references if the programmer forgot the functions name or parameters. You don’t have to remember the proper syntax to be a professional programmer; it is more important to understand a programming language’s fundamental principles and structures.
What is clean code? How can a developer write clean code and avoid sloppy code? This tutorial will give some basic principles for writing clean, readable C# code.
What is clean code?
To begin, we note there is no single definition of clean code. If you ask such a question to programmers, they will give their description and set of rules. Some points will overlap, and we can understand what clean code in C# is given these coincidences. Most developers believe clean code should meet these criteria:
- easy to read,
- clearly defined and documented,
- scales according to project needs,
- practical, and all redundant code (i.e., commented out code that will no longer be used under any circumstances) has been removed,
- follows specific best practices for the programming language, in this case, C#.
In other words, clean code in C# is easy to read, understand, maintain, and modify.
We have compiled a set of recommendations, which will help write more readable code so that it is understandable to all members of your team and specialists who will work on the project in the future.
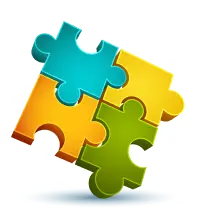
We are confident that we have what it takes to help you get your platform from the idea throughout design and development phases, all the way to successful deployment in a production environment!
Encapsulate conditionals
Encapsulation is a technique that helps isolate implementation details from the behavior provided to clients of another class. In addition, you may encapsulate conditional on providing better control of class members (reduce the possibility of you (or someone else) messing up the code). Thanks to this manipulation, the programmer may change one fragment of the clean code without affecting other parts.
Use private/protected members
Access modifiers are the particular keyword that C# uses to restrict access to class members to specify the scope of the members of the class. There are six access modifiers in C#:
- Public members are accessible anywhere inside or outside a class or assembly by creating an object of that class.
- Private members are limited to a class and not accessible outside that class, even by creating an object.
- Protected members are available within this class or in a class that is derived from it. But protected members cannot be accessed by creating an object of the class. Protected members can only be accessed through inheritance.
- Internal members are available anywhere in the assembly where the class resides. We can think of internals as a subset of publics, i.e., internals act like publics, but only in this assembly. They are not available outside this assembly.
- Protected Internal is a combination of Protected and Internal. Class members with this access modifier can be accessed in a derived class through inheritance or by creating an object or base class. But in a different assembly, this can only be accessed through the inheritance.
- Private Protected – such a modifier overcomes the limitations of Protected Internal. In the private protected access modifier, a derived class from another assembly cannot access members that are protected by Private.
You can utilize the protected accessibility modifier to help children access their parents’ properties. If you don’t use private/protected members (accidentally or intentionally), it may lead to errors in the code.
Don’t let your methods and classes get fat
When creating an application, we initially focus on the functional component. We don’t have rules to follow strictly; we put things where we want and make sure there are no functionality issues. As the application grows, things deteriorate, and it becomes difficult to manage the code. Growing business needs and poor architecture design will lead to further maintenance and testing problems. The optimal solution is moving towards a clean architecture. Don’t let your classes and methods do side work they were never meant to do.
Plan for application levels and clean code modularity. Follow the SOLID architecture principle when developing classes and methods. Each class should have one responsibility, and each method should perform one separate task. Things that have too many responsibilities are hard to maintain.
Avoid conditionals
At first glance, this may seem like an impossible task. You can use polymorphism to achieve the same task in many cases. Some programmers don’t understand why they have to avoid conditionals. The answer to the question lies in the concept of clean code in C#, which we talked about earlier: a function must only do one thing. When your classes and functions have if statements, you tell your users the function does more than one thing, polluting the code.
Try to use setters and getters
Properties in C# are a combination of fields and methods. They are not variables, so they cannot be passed as out or ref parameters in functions. Properties help check access to data, perform data validation before editing, and promote cleaner code as it avoids declaring explicit private variables with setters and getters.
The code for getters is executed when the value is read. Since it is a read procedure on a field, a getter must invariably return the result. A public getter means the property is readable by everyone. The private getter implies the property can only be read by the class and is, therefore, a write-only property.
The code for setters is executed when the value is written. The property can be assigned a value in its current form, or we may do some computations before giving it. On the one hand, a public setter means the value is editable by any object outside the class. On the other hand, it makes the property read-only and cannot be changed by others.
Handling Exceptions
There are some specific things to look out for when handling exceptions or when an exception occurs. Remember about such scenarios:
- Don’t throw exceptions in a final block, as this may hide previous exclusion in a try-catch block.
- Avoid non-public exclusion; all exceptions must be public.
- Don’t throw generic exceptions like Exception or SystemException.
- Breaking the debugging process may be due to explicitly throwing exceptions; call throws instead.
We recommend avoiding throwing exceptions from GetHashCode, ToString, static constructors, Object.Equals, implicit casts, and comparison operators
Use consistent capitalization
C# naming conventions are an essential part of C# coding standards and a good practice when you are developing a .NET applications. .NET naming conventions are standards of how the naming of variables, methods, classes, and other clean code elements should be defined.
To distinguish between words in an identifier, capitalize the first letter of each word in the identifier. Don’t use underscores to differentiate words or, for that matter, anywhere in identifiers. There are two appropriate ways to use consistent capitalization, depending on the usage of the identifier:
- Do utilize PascalCasing for all public member, type, and namespace names consisting of multiple words. Such convention capitalizes the first character of each word (including acronyms over two letters in length).
- We recommend using Camelcase notation for parameter names. In such a standard, two or more words are placed together without any space or underscore ( _ ), but the first letter of the first word is in lowercase, and the first letter of the next word is capitalized. Avoid using abbreviations and screaming capitalization, i.e., SAVEVALUE.
Most compound terms are treated as separate words for the goals of capitalization. Don’t capitalize each word in so-called closed-form compound words. These are compound words written as a single word, such as endpoint. Count the compound word in closed form as one word according to case guidelines. Use a dictionary to determine if a compound is written in a closed-form.
Refrain from using magic chains
Magic strings are string values that are specified in the code. They usually affect the code. In most cases, magic strings are virtual duplicates of themselves, and since they are updated automatically, they can be a source of errors.
In addition, the string may be associated with some form of external infrastructure or dependency, such as a configuration file name, a web URL, or a settings string. If the location of an external resource or how it is accessed changes, any magic lines referring to those resources need to be updated.
Pay attention to the changelogs
As technology changes, the programming language must also adapt. Indeed, like human languages, programming languages are constantly evolving and (mainly) improving. Because of this, a good developer will take note of the changelogs in their programming languages when a new version comes out. The central aspect of changelogs to pay attention to is deprecation. A deprecation in the changelog refers to a change to some language component that is currently deprecated but not prohibited. For example, Thread.Resume and Thread.Suspend are deprecated in C# because they can lead to deadlocks. However, technically they still exist in the language, and developers can use them.
As a rule, if some element is obsolete in the version of the language, it will be completely removed over time. When a new developer looks at your code, he doesn’t recognize an outdated piece of code you are typing, not to mention that the code will throw errors after it is updated.
The article covered only part of the recommendations to help write better and cleaner C# code. We hope you enjoyed it. Now get back to your work and make your code better!
Top Articles
ABC of DevOps Testing: Types, Best Practices, and Everything You Need to Know
I am here to help you!
Explore the possibility to hire a dedicated R&D team that helps your company to scale product development.
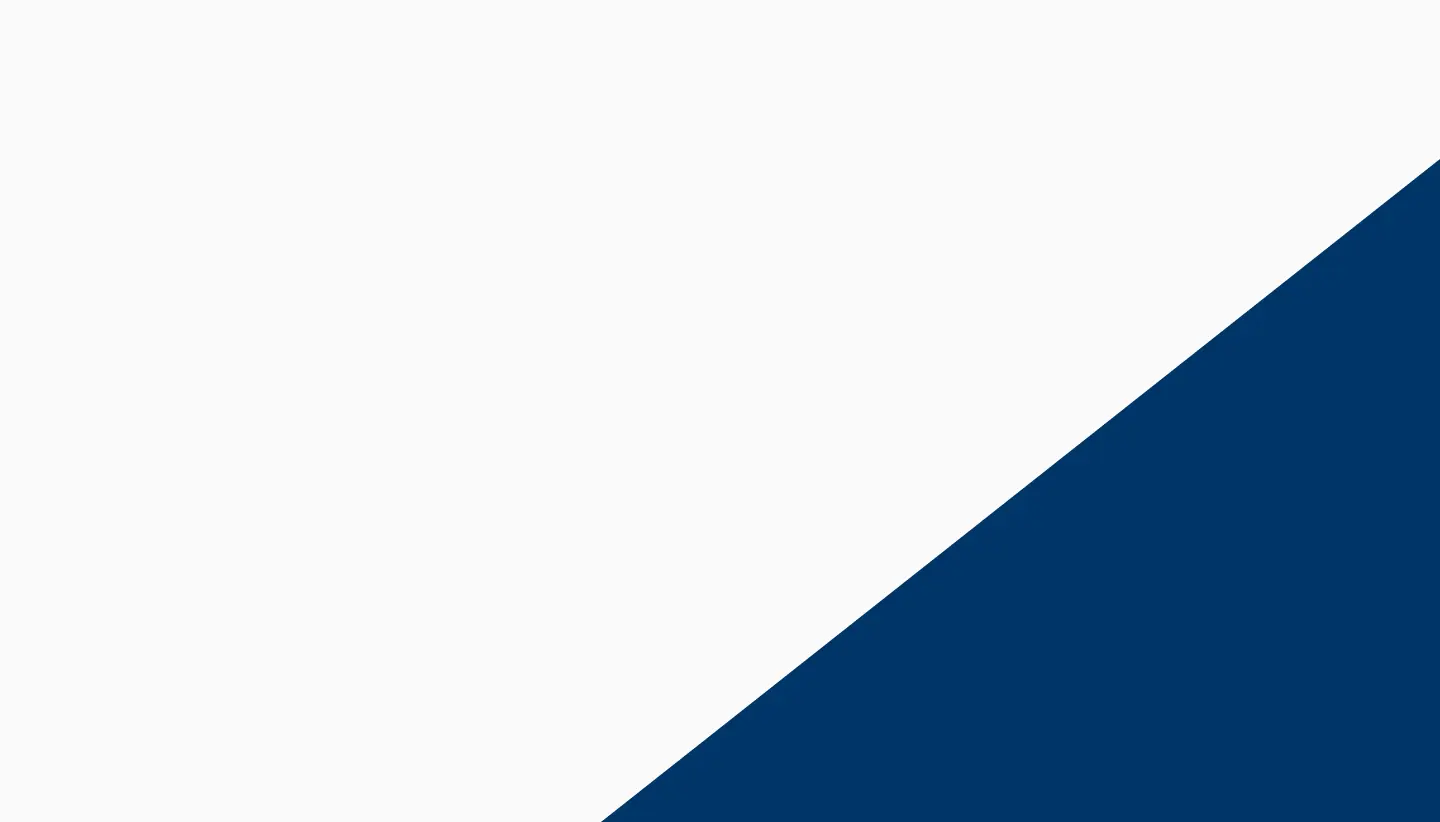
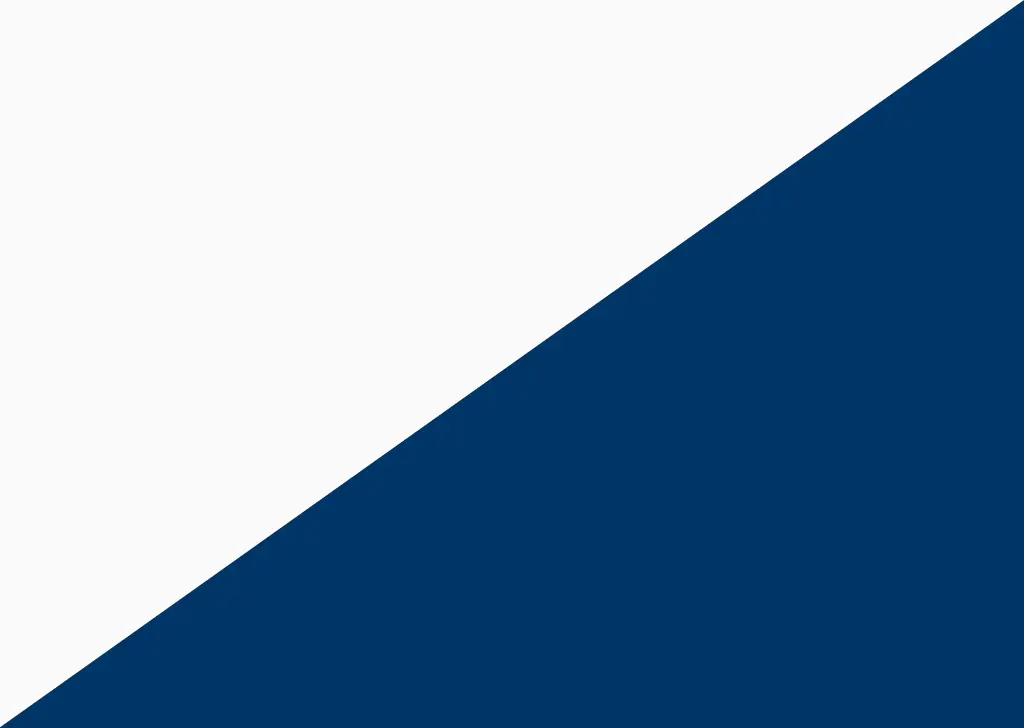